Introduction
This post will cover the creation of a performance testing framework using k6, as well as the steps involved in building and utilizing the framework.
setup
- Install k6 on your local machine by following the instructions provided in the documentation: https://k6.io/docs/get-started/installation/
- If you have homebrew installed, you can use the following command to install k6:
brew install k6
By completing these steps, you will have k6 properly set up and ready to use.
Framework Architecture
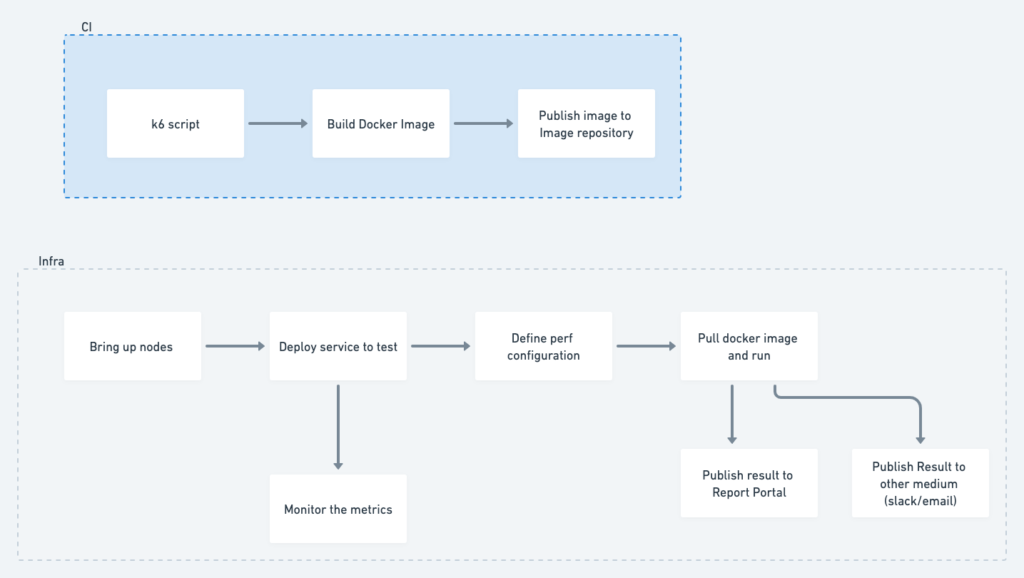
Sample Perf Script
import http from "k6/http"; import {check,fail,sleep} from "k6"; export let options = { stages:[ {"duration" : __ENV.STAGE_1_DUR, "target": __ENV.STAGE_1_VUS}, {"duration" : __ENV.STAGE_2_DUR, "target": __ENV.STAGE_2_VUS}, {"duration" : __ENV.STAGE_3_DUR, "target": __ENV.STAGE_3_VUS}, {"duration" : __ENV.STAGE_4_DUR, "target": __ENV.STAGE_4_VUS}, {"duration" : __ENV.STAGE_5_DUR, "target": __ENV.STAGE_5_VUS} ] } export default function(){ loadGoogleWebsite(); } function loadGoogleWebsite(){ let resp = http.get("https://www.google.com"); console.log("Response Status Code "+resp.status); check_and_fail(resp,200); } function check_and_fail(response,expectedStatusCode){ check(response,{"status code 200" : (res) => res.status === expectedStatusCode}) || fail("Expected "+expectedStatusCode+" status but obtained "+response.status); }
k6 run Google/googlebrowse.js
/\ |‾‾| /‾‾/ /‾‾/ /\ / \ | |/ / / / / \/ \ | ( / ‾‾\ / \ | |\ \ | (‾) | / __________ \ |__| \__\ \_____/ .io WARN[0000] `stages` was explicitly set to an empty value, running the script with 1 iteration in 1 VU execution: local script: Google/googlebrowse.js output: engine scenarios: (100.00%) 1 scenario, 1 max VUs, 10m30s max duration (incl. graceful stop): * default: 1 iterations for each of 1 VUs (maxDuration: 10m0s, gracefulStop: 30s) INFO[0000] Response Status Code 200 source=console ✓ status code 200 checks.........................: 100.00% ✓ 1 ✗ 0 data_received..................: 23 kB 65 kB/s data_sent......................: 639 B 1.8 kB/s http_req_blocked...............: avg=258.29ms min=258.29ms med=258.29ms max=258.29ms p(90)=258.29ms p(95)=258.29ms http_req_connecting............: avg=15.81ms min=15.81ms med=15.81ms max=15.81ms p(90)=15.81ms p(95)=15.81ms http_req_duration..............: avg=100.01ms min=100.01ms med=100.01ms max=100.01ms p(90)=100.01ms p(95)=100.01ms { expected_response:true }...: avg=100.01ms min=100.01ms med=100.01ms max=100.01ms p(90)=100.01ms p(95)=100.01ms http_req_failed................: 0.00% ✓ 0 ✗ 1 http_req_receiving.............: avg=516µs min=516µs med=516µs max=516µs p(90)=516µs p(95)=516µs http_req_sending...............: avg=631µs min=631µs med=631µs max=631µs p(90)=631µs p(95)=631µs http_req_tls_handshaking.......: avg=212.52ms min=212.52ms med=212.52ms max=212.52ms p(90)=212.52ms p(95)=212.52ms http_req_waiting...............: avg=98.86ms min=98.86ms med=98.86ms max=98.86ms p(90)=98.86ms p(95)=98.86ms http_reqs......................: 1 2.780852/s iteration_duration.............: avg=359.46ms min=359.46ms med=359.46ms max=359.46ms p(90)=359.46ms p(95)=359.46ms iterations.....................: 1 2.780852/s running (00m00.4s), 0/1 VUs, 1 complete and 0 interrupted iterations default ✓ [======================================] 1 VUs 00m00.4s/10m0s 1/1 iters, 1 per VU