There are situations where one workflow must be finished before another workflow can begin. An example of this is when we require a Docker image to be constructed before moving forward with the deployment of the image to the test automation environment and executing the suite of tests.
Workflow 1:
on: [ push ] jobs: cancel-previous-job: name: Cancel previous workflow if: always() timeout-minutes: 1 runs-on: ubuntu-latest steps: - name: Cancel previous workflow uses: styfle/cancel-workflow-action@0.9.1 if: github.ref != 'refs/heads/master' with: all_but_latest: true access_token: ${{ secrets.GITHUB_TOKEN }} workflow_id: ${{ github.event.workflow.id }} job1: name: Test Job 1 needs: [ cancel-previous-job ] runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2 - name: Run single command run: | sleep 30 echo "Job executed"
Workflow 2:
on: [ push ] jobs: cancel-previous-job: name: Cancel previous workflow if: always() timeout-minutes: 1 runs-on: ubuntu-latest steps: - name: Cancel previous workflow uses: styfle/cancel-workflow-action@0.9.1 if: github.ref != 'refs/heads/master' with: all_but_latest: true access_token: ${{ secrets.GITHUB_TOKEN }} workflow_id: ${{ github.event.workflow.id }} job2: name: Test Job 2s needs: [ cancel-previous-job ] runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2 - name: Get Build Image Workflow Status env: GITHUB_TOKEN: ${{ github.token }} BRANCH: ${{ github.event.pull_request.head.ref }} WORKFLOW: workflow_1.yml run: | echo "WORKFLOW_STATUS=$(sh ./.github/actions/status.sh)" >> $GITHUB_ENV - name: Check Workflow Status run: echo $WORKFLOW_STATUS
Script :
#!/bin/sh get_workflow_status_value() { URI="https://api.github.com" API_HEADER="Accept: application/vnd.github.v3+json" AUTH_HEADER="Authorization: token ${GITHUB_TOKEN}" branch_name=${BRANCH} workflow_file=${WORKFLOW} workflow_response=$(curl -sSD build_image_workflow_response_header.txt -H "${AUTH_HEADER}" -H "${API_HEADER}" "${URI}/repos/${GITHUB_REPOSITORY}/actions/workflows/$workflow_file/runs?branch=$branch_name&per_page=1" | jq '.') echo "$workflow_response" > build_image_workflow_response_body.txt cat build_image_workflow_response_header.txt >> build_image_workflow_log.txt cat build_image_workflow_response_body.txt >> build_image_workflow_log.txt workflow_details=$(echo "$workflow_response" | jq --raw-output '{conclusion: .workflow_runs[0].conclusion, status: .workflow_runs[0].status}') workflow_status=$(echo "$workflow_details" | jq --raw-output '.status') while [ "$workflow_status" != "completed" ] do sleep 1m workflow_response=$(curl -sSD build_image_workflow_response_header.txt -H "${AUTH_HEADER}" -H "${API_HEADER}" "${URI}/repos/${GITHUB_REPOSITORY}/actions/workflows/$workflow_file/runs?branch=$branch_name&per_page=1" | jq '.') echo "$workflow_response" > build_image_workflow_response_body.txt cat build_image_workflow_response_header.txt >> build_image_workflow_log.txt cat build_image_workflow_response_body.txt >> build_image_workflow_log.txt workflow_details=$(echo "$workflow_response" | jq --raw-output '{conclusion: .workflow_runs[0].conclusion, status: .workflow_runs[0].status}') workflow_status=$(echo "$workflow_details" | jq --raw-output '.status') done workflow_conclusion=$(echo "$workflow_details" | jq --raw-output '.conclusion') } get_workflow_status_value echo $workflow_conclusion
Example
Job 2 waits till Job 1 completes another workflow

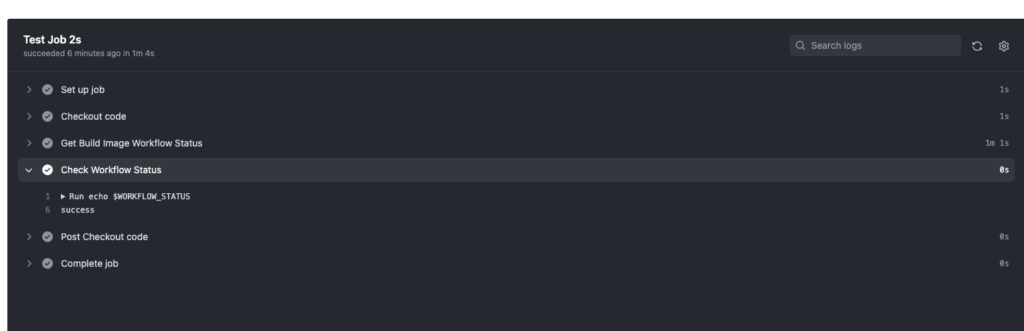